숙련 수업 목표
- 기획을 이해하고 파악하고 설계하기
1 기획 및 설계
DDD 기반 기획
- 전략적 설계
- Ubiquitous Language / Actor / Domain Event / Command / Policy / External System / Hotspot
- Aggregate : 비즈니스 로직 수행을 위한 객체의 집합 (주문 aggregate > 배송정보, 결제정보 domain model)
- Bounded context : Actor, Domain Event, Command 를 고려한 하나의 집합
- 전술적 설계
- Data 정의
- User - id / email / password / nickname / role
API (Application Programming Interface)
Application : 고유한 기능을 가진 모든 소프트웨어
Interface : 두 Application 간의 서비스 계약
웹 API
- 웹 서버와 웹 브라우저 간의 application interface
-모든 웹 서비스는 API 이지만 모든 API 가 웹 서비스는 아니다.
REST API (REpresentational State Transfer)
REST is not a protocol or a standard, it is an architectural style.
https://www.youtube.com/watch?v=RP_f5dMoHFc&t=861s
수강신청 API 설계해보기
Command | Method | URI |
회원가입 | POST | /signup |
프로필 수정 | PUT | /users/{userId}/profile |
로그인 | PUT | /login |
로그아웃 | PUT | /logout |
Course 생성 | POST | /courses |
Course 단건 조회 | GET | /courses/{courseId} |
Course 목록 조회 | GET | /courses |
Course 삭제 | DELETE | /courses/{courseId} |
Course 수정 | PUT | /courses/{courseId} |
Lecture 추가 | POST | /courses/{courseId}/lectures |
Lecture 단건 조회 | GET | /courses/{courseId}/lectures /{lectureId} |
Lecture 목록 조회 | GET | /courses/{courseId}/courses |
Lecture 수정 | PUT | /courses/{courseId}/lectures /{lectureId} |
Lecture 삭제 | DELETE | /courses/{courseId}/lectures/{lectureId} |
Course 신청 | PUT | /courses/{courseId}/applications |
Course 신청 내역 승인, 거절 | PATCH | /courses/{courseId}/applications/{applicationId} |
Course 신청 내역 단건 조회 | GET | /courses/{courseId}/applications/{applicationId} |
Course 신청 내역 목록 조회 | GET | /courses/{courseId}/applications |
Controller 및 DTO 작성해보기
Swagger 적용
springdoc 의 springdoc-openapi-starter-webmvc-ui 패키지를 dependencies 에 추가 -> 기본적으로 bean 등
implementation("org.springdoc:springdoc-openapi-starter-webmvc-ui:2.2.0")
Swagger 적용 후 로컬 8080 실행 화면
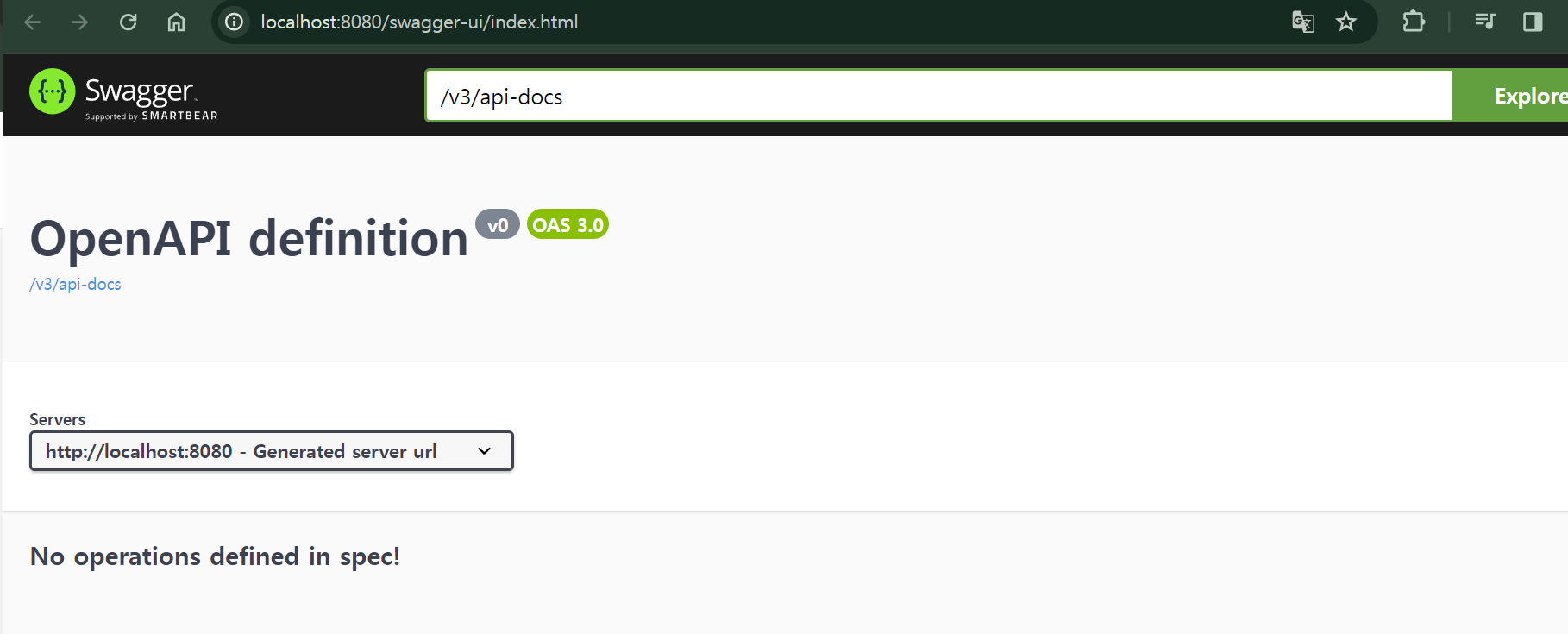
data 클래스
- 자동 생성 메소드 : toString(), hashCode(), equals()
data class CourseResponse(
val id: Long,
val title: String,
val description: String?,
val status: String,
val maxApplicants: Int,
val minApplicants: Int
)
fun main(args: Array<String>) {
val courseResponse = CourseResponse(
id = 1L,
title = "spring",
description = null,
status = "OPEN",
maxApplicants = 30,
minApplicants = 30
)
println(courseResponse.id)
println(courseResponse.title)
// data class 구조 분해 선언, 프로퍼티를 간단하게 변수에 할당 가능
val (id, title, description) = courseResponse
println(id)
println(title)
println(courseResponse.toString())
}
Handler Method
Controller 단에서 http의 요청을 처리하기 위해 지정하는 Annotation
@RequestMapping : 어떤 URI 을 담당하는지 알려줌, 하위에 지정된 URI가 추가됨
- @GetMapping
- @PostMapping
- @PutMapping
- @PatchMapping
- @DeleteMapping
@RestController : 주로 Data를 반환하는 데에 사용, @Controller 도 데이터를 반환하는 데 사용할 수 있지만 @RestController 가 일반적으로 RestFul서비스에서 데이터를 반환하기 위한 목적으로 사용됨.
@PathVariable : URI 경로 변수 값
@RequestBody : 클라이언트의 http 요청 body를 매개변수로 전달받을 때 JSON을 객체로 매핑
@RequestParam : HTTP 요청 파라미터 값을 매핑
@ResponseBody : Controller 메소드에서 반환되는 데이터를 http 규격에 맞는 값으로 반환
*ResponseEntity : 객체로 사용, 응답으로 변환될 정보를 모두 담은 요소들을 객체로 만들어서 반환
@RequestMapping("/courses/{courseId}/lectures")
@RestController
class LectureController {
@GetMapping
fun getLectureList(@PathVariable courseId: Long): ResponseEntity<List<LectureResponse>> {
TODO()
}
@GetMapping("/{lectureId}")
fun getLecture(
@PathVariable courseId: Long,
@PathVariable lectureId: Long
): ResponseEntity<LectureResponse> {
TODO()
}
@PostMapping("/{lectureId}")
fun addLecture(
@PathVariable courseId: Long,
@RequestBody addLectureRequest: AddLectureRequest //클라이언트가 전송한 데이터가 매핑됨
): ResponseEntity<LectureResponse> {
TODO()
}
@PutMapping("/{lectureId}")
fun updateLecture(
@PathVariable courseId: Long,
@RequestBody updateLectureRequest: UpdateLectureRequest
): ResponseEntity<LectureResponse> {
TODO()
}
@DeleteMapping("/{lectureId}")
fun deleteLecture(
@PathVariable courseId: Long,
@PathVariable lectureId: Long
): ResponseEntity<Unit> {
TODO()
}
}
API 와 DTO 작성에 반나절이 흘러갔다...
재밌다. 재밌는데 내가 이해가 느린거 아닌가 🥲🥲🥲🥲
오늘 http 책이 도착해서 원래 20시부터 읽으려고 했는데 🥲🥲
챌린지 반에 야심차게 도전했다
다른 사람들이 관심 있는 것 다~처음 들어본다 ! 세션 전까지 꼭 다 알아들을 수 있도록 🥲🥲
JPA
CI/CD
성능테스트
데이터 모델링
구조 잡기
확장 가능한 코드
테스트 코드
함수형 프로그래밍
디자인 패턴
Spring security
아키텍쳐
'왕초보일지' 카테고리의 다른 글
231221 TIL | (2) | 2023.12.21 |
---|---|
231220 TIL | Spring Service Layer 작성 (1) | 2023.12.20 |
키오스크 피드백 반영하기 (0) | 2023.12.18 |
231218 TIL Spring 입문 1주차 강의 (1) | 2023.12.18 |
231215 TIL (1) | 2023.12.15 |